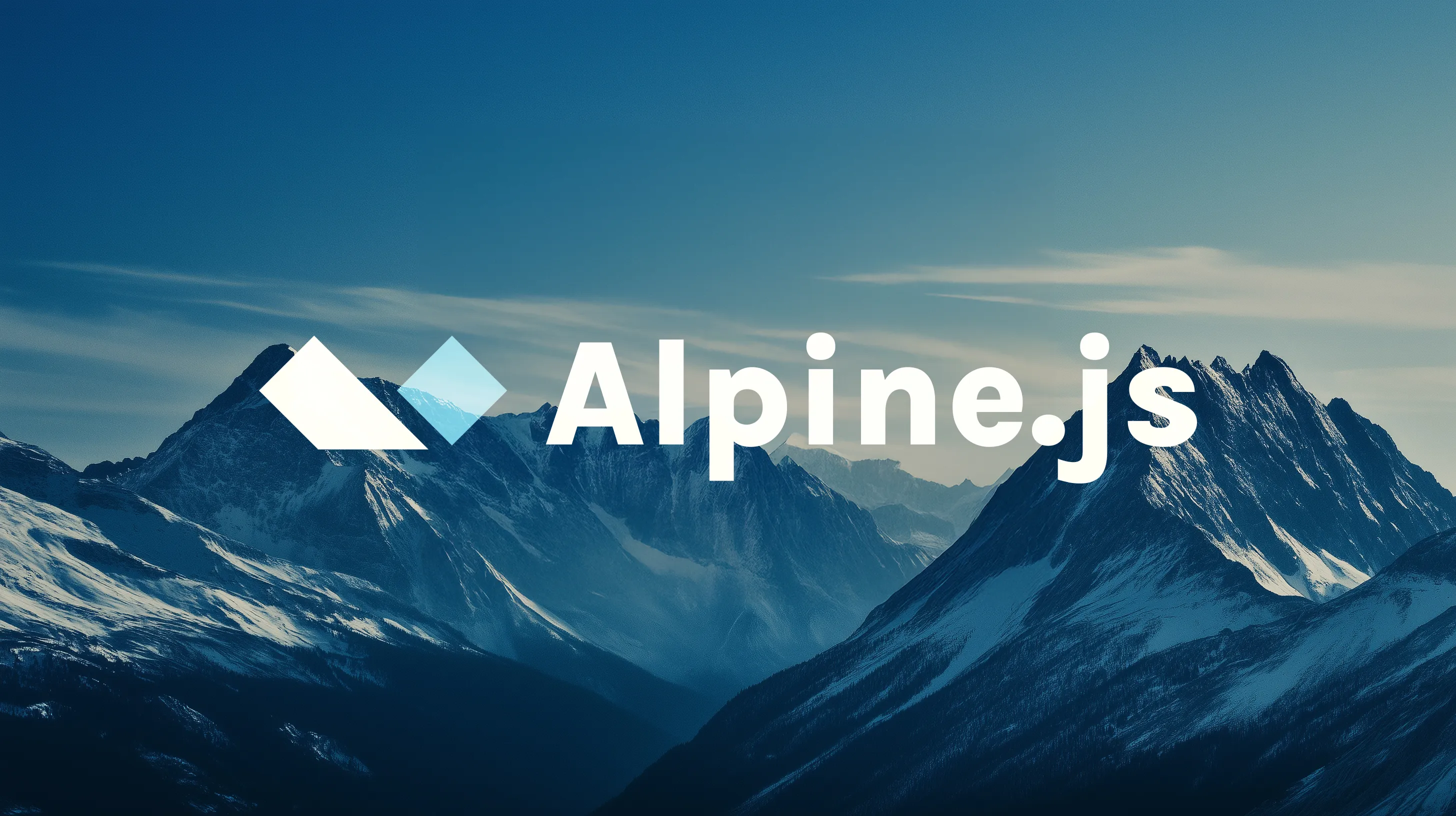
Table of Contents
Share
What is AlpineJS and Why Should You Use It?
AlpineJS is a lightweight and fast JavaScript library designed to add dynamic and interactive features to modern web applications. It offers the power of larger frameworks like Vue.js or React but in a much simpler and lighter way. Because of these features, it is often preferred for smaller projects or applications that do not require complex frameworks. With its HTML-centric structure, AlpineJS provides a balanced solution between simplicity and flexibility.
Advantages of AlpineJS
- Lightweight and Fast: Its small file size allows it to load quickly and improve performance.
- HTML-Centric Approach: Most of the JavaScript code can be controlled directly through HTML elements.
- Easy Integration: It is easy to learn and implement, making it accessible to anyone with basic JavaScript knowledge.
Harnessing the Power of Directives: Core Concepts of AlpineJS
The concept of directives is one of the fundamental building blocks of AlpineJS. Directives add specific behavior to an HTML element and are defined with the x-
prefix. These directives offer an HTML-based coding structure and make implementing dynamic solutions straightforward.
Popular Directives
x-data:
Defines the data model for components.x-bind:
Dynamically binds attributes to HTML elements.x-show:
Controls the visibility of elements.x-model:
Enables two-way data binding with form elements.
With directives, you can easily add interactive features without writing complex JavaScript code.
Phone Number Masking with AlpineJS and IMask
Formatting phone number inputs to match a standard format significantly improves the user experience. Using the IMask library, you can implement a phone number mask that complies with the Turkish format.
1. Installing IMask and AlpineJS
First, install the IMask and AlpineJS libraries:
Installing IMask:
npm install imask
Installing AlpineJS:
npm install alpinejs
2. Creating a Custom AlpineJS Directive
To create a phone number mask for Turkey, you can define a custom directive. Below is an example implementation:
import Alpine from 'alpinejs';
import IMask from 'imask';
// Define the 'phone' directive
Alpine.directive('phone', (el, { expression }, { Alpine }) => {
const maskOptions = {
mask: '+9\0 (500) 000 0000', // Turkish phone format
lazy: false // Always display the mask
};
const mask = IMask(el, maskOptions).on('accept', () => {
// Assign the unmasked value (5XXXXXXXXX) to the corresponding object
evaluate(`${expression} = '${mask.unmaskedValue}'`)
});
});
window.Alpine = Alpine;
Alpine.start();
3. Usage
Once the directive is defined, you can use it in HTML as follows:
<div x-data="{ form: { phone: '' } }">
<input
type="text"
name="phone"
class="input-field"
x-phone="form.phone"
required
>
</div>
Conclusion and Evaluation
With this method, you can enhance the user experience for phone number inputs while ensuring data accuracy. The data collected from the user is stored in an unmasked format in the backend, making form processing more efficient. This solution can also be customized for different data types, limited only by your imagination.
If you'd like to learn more or develop similar solutions for your projects, feel free to reach out to us. We'd be happy to assist you!
“Writing is seeing the future.” Paul Valéry