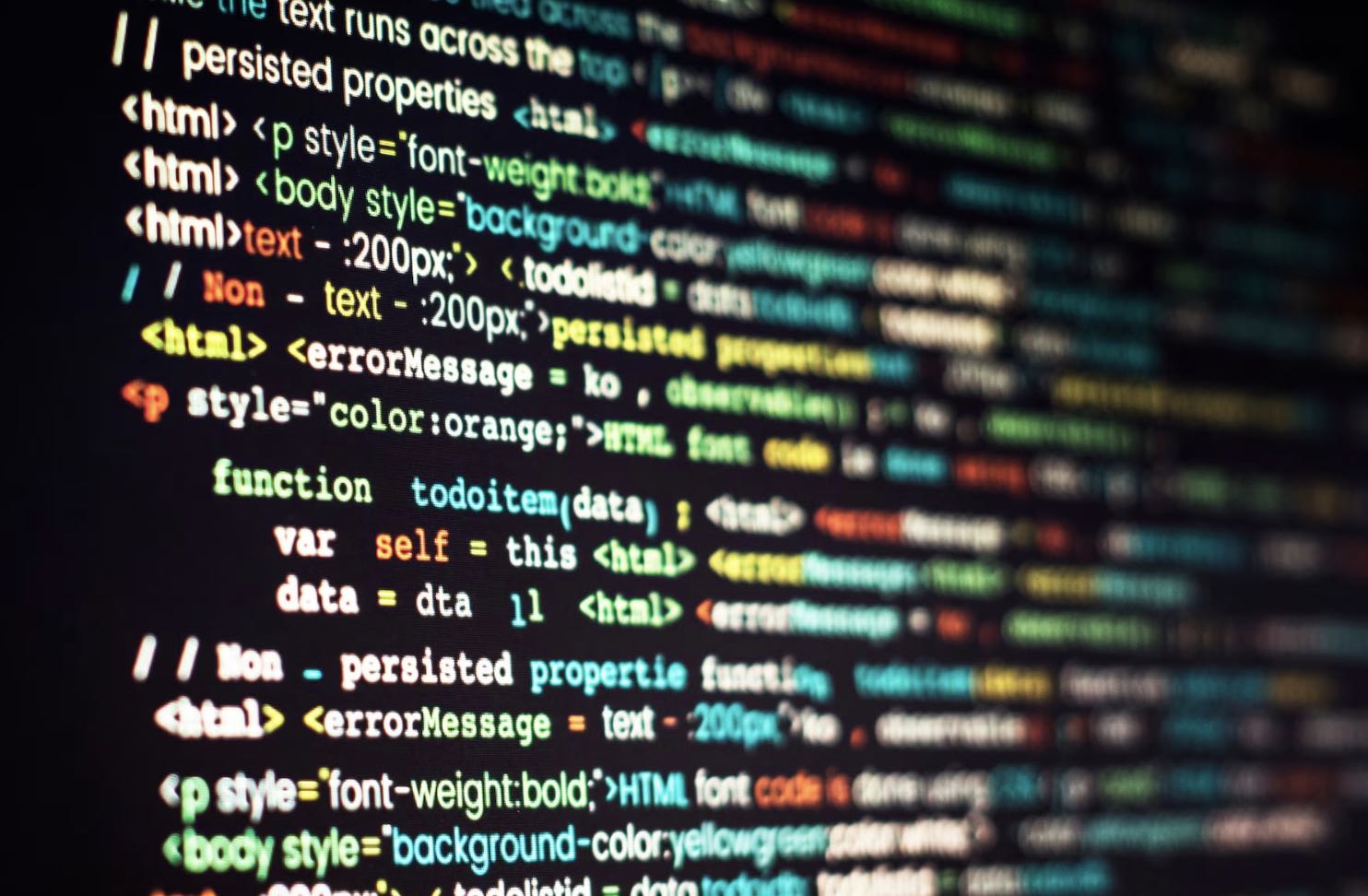
Table of Contents
- What is Rust?
- The Ownership Model in Rust
- Static Typing and Performance Optimization in Rust
- Error Handling Mechanisms in Rust
- Why is Rust So Popular Among Developers?
- Rust vs C++: A Comparison of Two Systems Programming Languages
- Notable Tools and Libraries in the Rust Ecosystem
- Applications of Rust Across Different Domains
- Learning Resources for Aspiring Rustaceans
- Community Support & Events in the World of Rust
- Challenges Faced When Working with Rust
- Conclusion
Share
In recent years, Rust has emerged as one of the most beloved programming languages in the developer community. In fact, it has consistently ranked at the top of Stack Overflow's "most loved language" survey for multiple years. But what exactly is Rust, and why has it gained such popularity? In this article, we will explore what Rust is, why it stands out from other programming languages, and how it addresses key challenges faced by developers today.
What is Rust?
Rust is a systems programming language designed for performance, safety, and concurrency. It was initially developed by Mozilla Research and officially launched in 2010. With Rust, developers can build software that is both fast and reliable, thanks to its unique features like the ownership model and borrow checker. These mechanisms ensure memory safety without needing a garbage collector, distinguishing Rust from languages like C++ and Python.
One of the key reasons for Rust’s popularity is how it addresses memory safety issues in systems programming, where languages like C and C++ fall short. By integrating modern development principles, Rust offers a more secure alternative for systems programming while maintaining high performance.
Example: A Simple Rust Program
fn main() {
println!("Hello, world!");
}
This simple Rust program prints "Hello, world!" to the console. Even though it's basic, this example illustrates how Rust's syntax is both straightforward and similar to other popular programming languages.
The Ownership Model in Rust
One of the most significant features of Rust is its ownership model, which is at the core of its memory safety features. In Rust, each value has a single owner, and the ownership is tracked at compile time. When the owner goes out of scope, Rust automatically deallocates the memory. This approach eliminates common memory issues like null pointer dereferencing and data races.
Rust also employs a borrow checker, which ensures that data is either borrowed immutably (for reading) or mutably (for writing), but never both simultaneously. This mechanism prevents data races at compile time.
Code Block: Ownership and Borrowing in Rust
fn main() {
let s1 = String::from("Hello");
let s2 = &s1; // Borrowing immutably
println!("{}", s2);
}
In this code snippet, s2
borrows the string s1
without taking ownership of it. Rust ensures that s1
remains valid and prevents any modifications while it is borrowed.
Static Typing and Performance Optimization in Rust
Rust is a statically typed language, meaning that types are checked at compile time. This allows for improved performance optimization, as the Rust compiler can make assumptions about the types and memory usage upfront. With static typing, developers can catch potential bugs earlier in the development cycle, making the codebase more reliable.
Rust optimizes performance through its fine-grained control over memory. By eliminating runtime garbage collection, Rust achieves the performance benefits traditionally associated with C and C++ but without the associated memory safety risks.
Code Block: Static Typing Example
fn add(a: i32, b: i32) -> i32 {
a + b
}
fn main() {
let result = add(5, 10);
println!("The result is: {}", result);
}
This example showcases Rust’s static typing. The function add
takes two integers and returns their sum. The static typing ensures that the correct types are used throughout the function.
Error Handling Mechanisms in Rust
In Rust, error handling is explicit, and it encourages developers to handle potential failures early and gracefully. Rust introduces two primary types for error handling: Result and Option.
- Result: Used for functions that might return an error.
- Option: Used for values that may or may not exist (similar to nullable types in other languages).
This approach provides more control over how errors are handled and makes the code more resilient.
Code Block: Error Handling Example with Result
fn divide(dividend: f64, divisor: f64) -> Result<f64, String> {
if divisor == 0.0 {
Err(String::from("Cannot divide by zero"))
} else {
Ok(dividend / divisor)
}
}
fn main() {
match divide(10.0, 2.0) {
Ok(result) => println!("Result: {}", result),
Err(err) => println!("Error: {}", err),
}
}
This example demonstrates Rust's Result type in action. The divide
function returns a Result
, either an Ok
with the result or an Err
if an invalid operation occurs (such as dividing by zero).
Why is Rust So Popular Among Developers?
There are several reasons why Rust has gained so much popularity among developers. According to surveys like Stack Overflow's, developers appreciate Rust for its:
- Memory safety: No garbage collector, but also no risk of null pointers or dangling references.
- Performance: Comparable to C++ but with more safety guarantees.
- Concurrency support: Rust’s design makes it easier to write safe concurrent programs, something traditionally difficult in C and C++.
- Modern toolchain: Tools like Cargo simplify package management and project setup.
Rust vs C++: A Comparison of Two Systems Programming Languages
Rust and C++ are both powerful systems programming languages, but they take different approaches to safety and performance. While C++ allows for manual memory management, Rust automates memory safety through its ownership model, preventing common memory bugs at compile time.
Key Differences:
- Memory Safety: Rust has built-in safety features like ownership and borrowing, while C++ relies on manual memory management.
- Concurrency: Rust has built-in mechanisms to prevent data races, making concurrent programming safer compared to C++.
Notable Tools and Libraries in the Rust Ecosystem
The Rust ecosystem offers powerful tools that make development easier:
- Cargo: The package manager and build system for Rust, responsible for managing dependencies and building projects.
- crates.io: The official Rust package registry where developers can find and publish libraries.
- rustfmt: A tool for automatically formatting Rust code to keep it consistent.
- Clippy: A linter that helps catch common mistakes and improve the quality of Rust code.
Applications of Rust Across Different Domains
Rust is used in a wide range of domains, including:
- Backend systems: Rust powers reliable backend systems due to its performance and safety.
- Web development: Frameworks like Rocket and Actix make Rust a strong choice for web development.
- Embedded systems: Rust's performance characteristics make it suitable for low-level programming, such as in embedded systems.
Learning Resources for Aspiring Rustaceans
For those looking to learn Rust, there are a variety of resources available:
- The Rust Book: Official documentation that is beginner-friendly and comprehensive.
- Rustlings: A collection of small exercises that teach Rust syntax and concepts.
- Rust by Example: A detailed guide with practical examples to understand Rust.
Community Support & Events in the World of Rust
The Rust community is highly active, with many events and conferences such as RustConf and RustFest. These events foster collaboration and innovation within the Rust ecosystem, making it easier for developers to stay up to date and connected.
Challenges Faced When Working with Rust
Despite its many advantages, Rust can be challenging for newcomers. The strict compile-time checks can be frustrating, and some developers may find the syntax difficult to grasp at first. Additionally, while the ecosystem is growing, certain libraries and tools are still maturing.
Conclusion
Rust is a powerful and modern programming language that addresses key pain points in systems programming, such as memory safety, concurrency, and performance. Despite the challenges that come with learning Rust, the benefits it offers—such as safer code and excellent performance—make it one of the best programming languages to learn today. Whether you're developing backend systems, web applications, or embedded systems, Rust provides a reliable and efficient solution.
Is Rust better than Python?
Whether Rust is better than Python depends on the context of use. Rust is a systems programming language known for its performance and memory safety, making it ideal for low-level programming, embedded systems, and performance-critical applications. On the other hand, Python is a high-level language favored for its ease of use, readability, and extensive libraries, making it suitable for web development, data analysis, and scripting. If performance and safety are your primary concerns, Rust may be the better choice; however, for rapid development and versatility, Python excels.
What language is Rust used for?
Rust is primarily used for systems programming, where performance and safety are crucial. It is commonly employed in developing operating systems, web browsers, and game engines. Additionally, Rust has gained traction in web development through frameworks like Rocket and Actix, as well as in embedded systems programming due to its low-level control over hardware. The language's ability to handle concurrent tasks safely also makes it suitable for high-performance applications.
Is Rust a dying language?
No, Rust is not a dying language. In fact, it is experiencing significant growth in popularity. According to the Stack Overflow Developer Survey, Rust has consistently ranked as one of the most loved programming languages. Its active community, robust ecosystem, and ongoing development ensure that it continues to thrive. Many companies are adopting Rust for critical projects due to its safety and performance features.
Is Rust basically C++?
While Rust and C++ share similarities as systems programming languages, they are not the same. Both offer high performance and low-level memory control, but Rust provides built-in features like the ownership model and borrow checker to ensure memory safety and prevent data races. C++, on the other hand, requires manual memory management and lacks some of the safety guarantees that Rust offers. Therefore, while they may serve similar purposes, Rust's design philosophy prioritizes safety and concurrency in a way that C++ does not.
“Writing is seeing the future.” Paul Valéry