Blog / Development
Securing Your Firestore Data with Custom Token Authentication in Laravel and Firebase
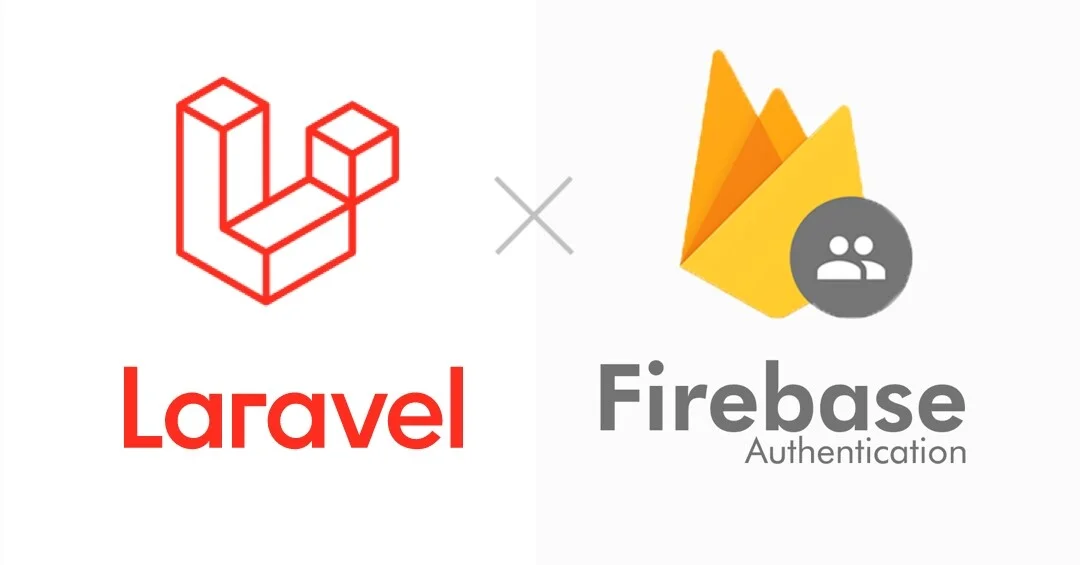
Introduction
Using Laravel and Firebase, we will create a custom token and perform authentication with this token.
Before we begin, Firebase Admin SDK for PHP and Javascript SDK for client should be installed in your application.
Our Scenario:
As you know, we need to define rules for the security of the data stored in Firestore, otherwise our data will be accessible to anyone with the right information. In order to define the rules, we need to use Firebase's own authentication system.
In this case, we will be able to write an isolated authentication system only for the data we store in firestore, without mixing it with the authentication system we keep in Laravel.
For this case, we will try to perform auth transactions with Custom Token.
We will need to create the relevant custom token on the Laravel side and provide it to the client side.
Initializing the Auth component
First of all, we need to initialize Firebase auth helper to creating custom token:
$auth = app('firebase.auth');
Create Custom Token
The Firebase Admin SDK includes a feature for creating custom tokens. To do this, you'll need to provide a userId (UID) on your Laravel authentication system as a minimum requirement. This UID can be any string, but it should be able to clearly distinguish the user or device being authenticated. Keep in mind that these tokens will automatically expire after one hour.
// Get your authenticated user id with auth helper or Auth::id() facade.
$uid = auth()->id();
$customToken = $auth->createCustomToken($uid);
return $customToken->toString();
This code will generate custom token to using on your firebase app.
Sign In With Custom Token
Now, we had custom token. After that, we need to provide that token to client side for sign in your firebase app.
Before that, as mentioned in Introduction section you have to installed Javascript SDK for client for Firebase SDK.
import { getAuth, signInWithCustomToken } from "firebase/auth";
const yourSignInWithCustomTokenFunction = async () => {
try {
// get your custom token from backend.
const { data } = await generateFirebaseSignInToken();
const customToken = data.generateFirebaseSignInToken;
const auth = getAuth();
const userCredential = await signInWithCustomToken(auth, customToken);
// Signed in
const user = userCredential.user;
} catch (error) {
console.error("Error:", error);
}
};
// call yourSignInWithCustomTokenFunction anywhere you want in your app.
yourSignInWithCustomTokenFunction();
Now you can check user signed in using that token on Firebase Cloud (Authentication).
Add Rules to Firestore Database
Now we are ready to add some rules to our database. It means, we can secure our data privacy by that rules to someone else cannot read it. Here's example rule:
rules_version = '2';
service cloud.firestore {
match /databases/{database}/documents {
match /{document=**} {
allow read: if request.auth != null;
allow write: if false;
}
}
}
This rules allowed to read data just for authenticated users.
Conclusion
In our Laravel application, we leverage the Firebase Admin SDK to generate custom tokens. Subsequently, we furnish these custom tokens to our client-side applications, enabling them to utilize these tokens for authentication within the Firebase authentication system.
In short, our backend system handles the token creation and verification within our Laravel application and Client side app. This approach lets you log in to the Firebase app using these tokens, providing a secure and user-friendly authentication process. Enjoy the benefits it offers!
“Writing is seeing the future.” Paul Valéry