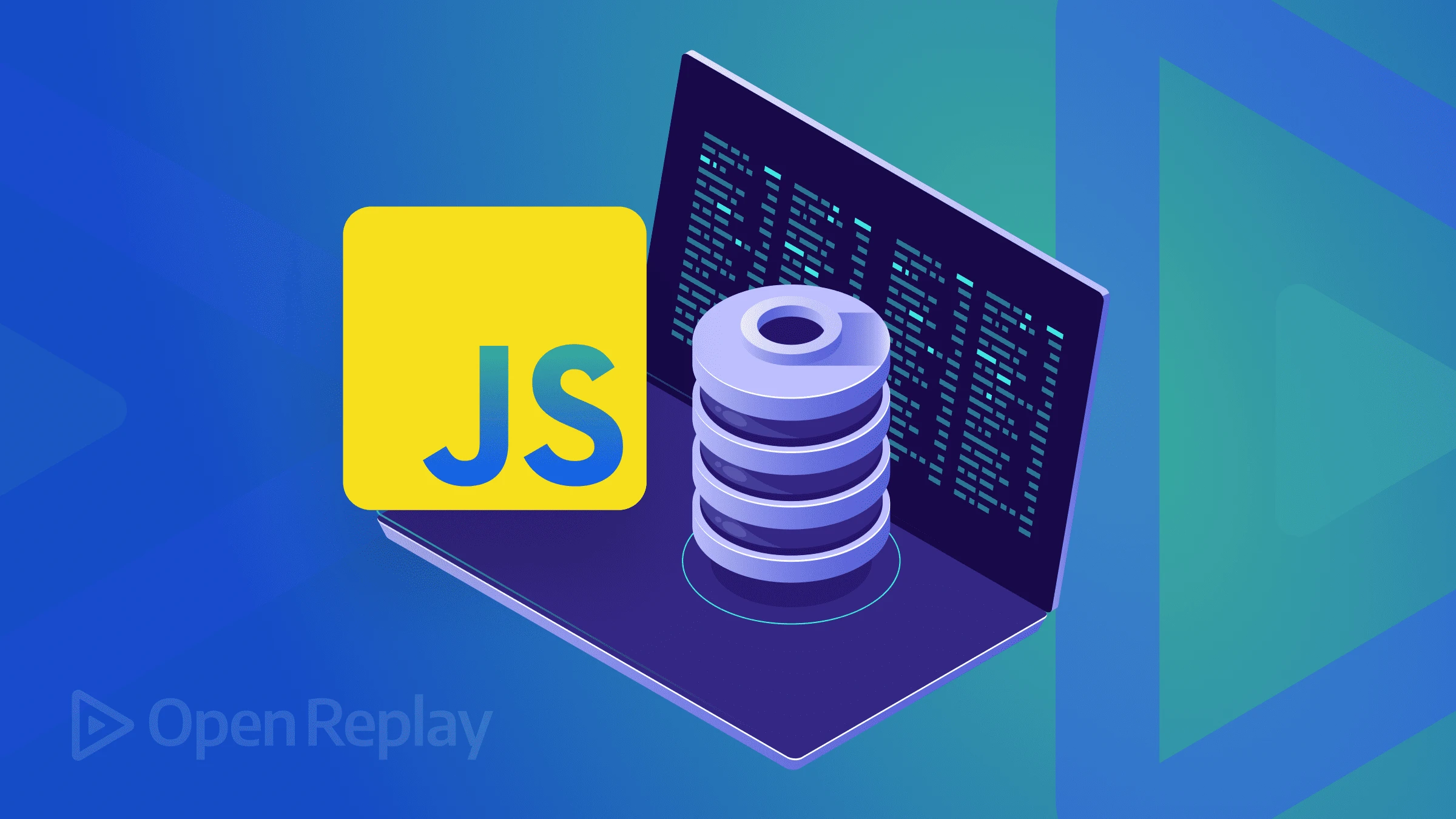
In the world of software development, JSON Web Tokens (JWT) have become an industry standard (RFC 7519) for securely representing claims between parties. JWTs are ubiquitous today, but a key question arises: Where should we store these tokens on the front end?
In this article, we'll delve into the two common options for storing tokens: Cookies and Local Storage.
A Comparative Analysis
Local Storage
Local Storage provides a straightforward approach to token storage, as it involves using the localStorage object:
localStorage.setItem("yourTokenName", yourToken);
localStorage.getItem("yourTokenName", yourToken);
Pros:
Convenience: Local Storage eliminates the need for a backend setup; you can handle it with pure JavaScript.
Ample Storage: With a capacity of approximately 5MB, Local Storage can accommodate substantial data.
Cons:
XSS Vulnerability: Local Storage is susceptible to Cross-Site Scripting (XSS) attacks. Malicious actors can exploit JavaScript vulnerabilities to access and extract access tokens stored in Local Storage.
Cookies
Managing cookies involves setting them through code or HTTP requests:
document.cookie = "cookieName=value";
// or via HTTP header
Set-Cookie: <cookie-name>=<cookie-value>
Pros:
Enhanced Security: Utilizing httpOnly and secure cookies prevents JavaScript access. This adds a layer of security against attackers attempting to read access tokens from cookies.
Expiry Control: Cookies can be configured with an expiration date, ensuring token validity for a specific duration.
Cons:
Limited Storage: Cookies offer only 4KB of storage space.
Security Considerations: While more secure than Local Storage, cookies are not entirely immune to security concerns.
Security
XSS Attacks
As highlighted, Local Storage's susceptibility to XSS attacks is a significant concern. Exploiting JavaScript vulnerabilities, attackers can compromise access tokens stored in Local Storage. However, while httpOnly cookies prevent direct JavaScript access, they are not completely impervious to XSS attacks targeting access tokens.
Attackers can still send HTTP requests to the server, leveraging the automatic inclusion of cookies. Although this approach is less straightforward, it demonstrates that cookies are not entirely immune to XSS-related token theft.
CSRF Attacks
Cross-Site Request Forgery (CSRF) is a vulnerability that coerces users into unintended actions. This risk can be mitigated by incorporating the sameSite flag in cookies and integrating anti-CSRF tokens.
Conclusion
In the ongoing debate between Cookies and Local Storage for token storage, cookies emerge as the more preferable choice, albeit not without vulnerabilities. The reasons are as follows:
-
Reduced XSS Vulnerability: Although both storage options face XSS threats, httpOnly cookies provide stronger protection against attackers attempting to exploit JavaScript vulnerabilities.
-
Mitigating CSRF Attacks: The inclusion of sameSite flags and anti-CSRF tokens significantly alleviates the risk of CSRF attacks when using cookies.
Ultimately, token storage hinges on balancing security with functionality. Cookies, despite their limitations, prove to be a more secure option, particularly when combined with precautionary measures. Whether dealing with larger tokens or utilizing specific headers, cookies can accommodate these demands while upholding a higher level of security.
“Writing is seeing the future.” Paul Valéry